سوال: برنامه ای برای نمایش اطلاعات دانش آموز بنویسید. برای نوشتن این برنامه کارهای زیر را انجام بدهید:
• کلاسی برای دانش آموز شامل ویژگی های فردی )کد دانش آموز نام نام خانوادگی نام پدر( و نمرات 3 درس دانش آموز تعریف کنید.
• سه متد سازنده، تعریف کنید که متد اول مقدار یک نمره، متد دوم مقدار دو نمره و متد سوم مقدار سه نمره را تعیین کند.
• متدی در کلاس قرار دهید که مقدار برگشتی آن، معدل این سه نمره باشد.
• از برچسب برای نمایش ویژگی های فردی، نمره ها و معدل و اطلاعات دانش آموز برروی فرم استفاده کنید. برای ایجاد شیء مربوط به هر دانش آموز، از یکی از متدهای سازنده استفاده نمایید.
حل مساله :
ابتدا رو نام پروژه کلیک راست میکنیم و سپس بر روی Add و Class کلیک مینماییم
نام کلاس را studentInfo درج میکنیم.کلاس مورد نظر بصورت زیر میباشد.
class studentInfo
{
int studentCode;
string studentFname, studentLname, studentFatherName;
double studentProgramingPoint, studentMathPoint, studentHistoryPoint;
public studentInfo(double programingPoint)
{
studentProgramingPoint = programingPoint;
}
public studentInfo(double programingPoint, double mathPoint)
{
studentProgramingPoint = programingPoint;
studentMathPoint = mathPoint;
}
public studentInfo(double programingPoint, double mathPoint, double historyPoint)
{
studentProgramingPoint = programingPoint;
studentMathPoint = mathPoint;
studentHistoryPoint = historyPoint;
}
public int StudentCode
{
get { return studentCode; }
set { studentCode = value; }
}
public string StudentFatherName
{
get { return studentFatherName; }
set { studentFatherName = value; }
}
public string StudentLname
{
get { return studentLname; }
set { studentLname = value; }
}
public string StudentFname
{
get { return studentFname; }
set { studentFname = value; }
}
public double StudentHistoryPoint
{
get { return studentHistoryPoint; }
set { studentHistoryPoint = value; }
}
public double StudentMathPoint
{
get { return studentMathPoint; }
set { studentMathPoint = value; }
}
public double StudentProgramingPoint
{
get { return studentProgramingPoint; }
set { studentProgramingPoint = value; }
}
}
فرم برنامه را بصورت زیر طراحی میکنیم
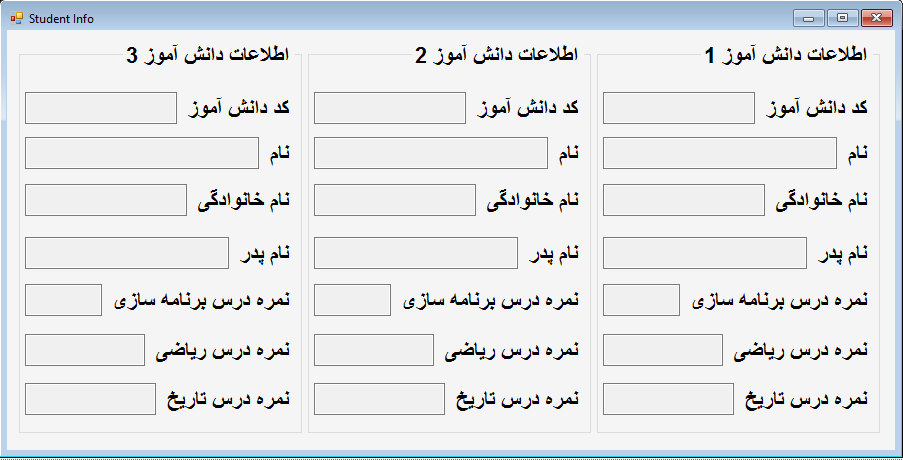
سپس در رویداد load فرم کدهای زیر را مینویسیم.
//Student 1:
studentInfo s1 = new studentInfo(19.50, 17.00, 13.25);
s1.StudentCode = 1;
s1.StudentFname = "Hasan";
s1.StudentLname = "Hasani";
s1.StudentFatherName = "Reza";
txtStudentCode1.Text = s1.StudentCode.ToString();
txtStudentFname1.Text = s1.StudentFname;
txtStudentLname1.Text = s1.StudentLname;
txtStudentFatherName1.Text = s1.StudentFatherName;
txtProgramingPoint1.Text = s1.StudentProgramingPoint.ToString();
txtMathPoint1.Text = s1.StudentMathPoint.ToString();
txtHistoryPoint1.Text = s1.StudentHistoryPoint.ToString();
//Student 2:
studentInfo s2 = new studentInfo(19,14);
s2.StudentCode = 2;
s2.StudentFname = "Hosein";
s2.StudentLname = "Hoseini";
s2.StudentFatherName = "Ali";
txtStudentCode2.Text = s2.StudentCode.ToString();
txtStudentFname2.Text = s2.StudentFname;
txtStudentLname2.Text = s2.StudentLname;
txtStudentFatherName2.Text = s2.StudentFatherName;
txtProgramingPoint2.Text = s2.StudentProgramingPoint.ToString();
txtMathPoint2.Text = s2.StudentMathPoint.ToString();
txtHistoryPoint2.Text = s2.StudentHistoryPoint.ToString();
//Student 3:
studentInfo s3 = new studentInfo(18);
s3.StudentCode = 3;
s3.StudentFname = "Mohammad";
s3.StudentLname = "Mohammadi";
s3.StudentFatherName = "Sajjad";
txtStudentCode3.Text = s3.StudentCode.ToString();
txtStudentFname3.Text = s3.StudentFname;
txtStudentLname3.Text = s3.StudentLname;
txtStudentFatherName3.Text = s3.StudentFatherName;
txtProgramingPoint3.Text = s3.StudentProgramingPoint.ToString();
txtMathPoint3.Text = s3.StudentMathPoint.ToString();
txtHistoryPoint3.Text = s3.StudentHistoryPoint.ToString();
در پایان پس از اجرای برنامه خروجی به شکل زیر خواهد بود
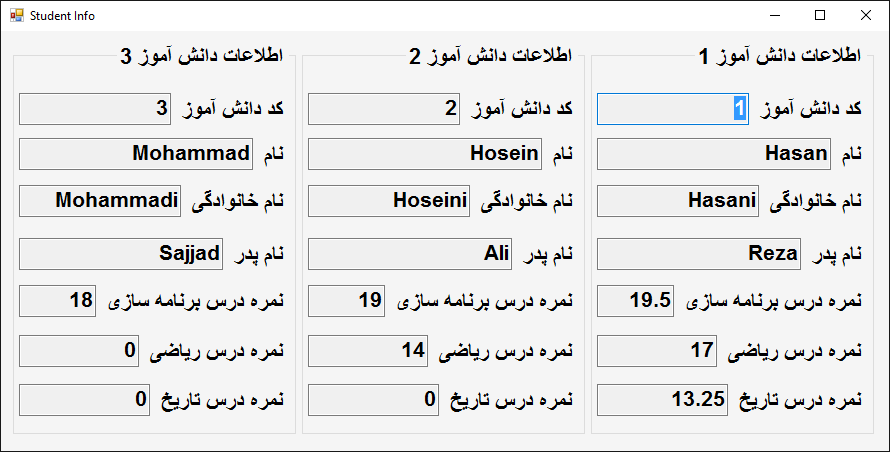
این برنامه توسط دانش آموز رضا سعیدی نژاد ارسال شده است